Okay, so today I’m gonna walk you through how I tackled a simple but kinda neat task: getting a list of MLB teams in alphabetical order. Sounds easy, right? Well, it was, but let me show you how I did it step-by-step.
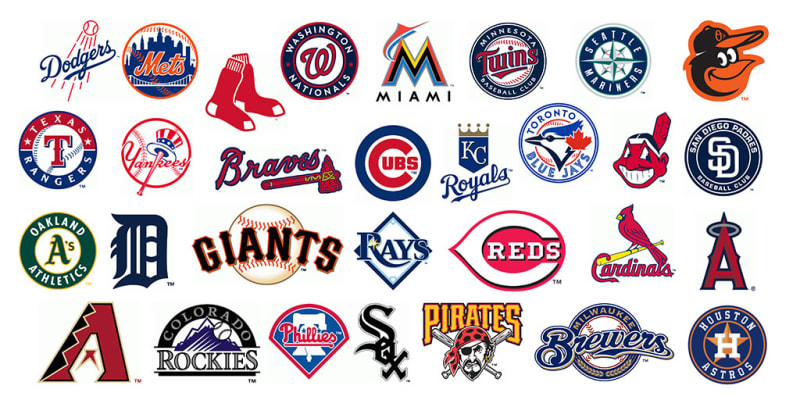
First things first, I needed to grab the data. I didn’t wanna type all the team names myself – that’s just asking for trouble. So, I searched around and found a decent list of MLB teams online. I copied that list into a plain text file. Nothing fancy, just a list with each team on a new line.
Next, I fired up my Python interpreter. This is where the fun begins! I started by reading the text file. I used the `open()` function to open the file, and then read all the lines into a list. Each line in the list was a team name.
But here’s the thing: each team name had a newline character at the end (`n`). That’s a no-go for sorting. So, I used a list comprehension to strip those pesky newline characters off. It looked something like this: `team_names = [*() for team in team_names]`. Simple, but effective!
Now that I had a clean list of team names, I used Python’s `sorted()` function to sort them alphabetically. This function is super easy to use. I just passed it my list of team names, and it returned a new list with the teams sorted alphabetically.
Finally, I printed out the sorted list. I used a simple `for` loop to iterate through the sorted list and print each team name to the console.
And that’s it! I had a list of MLB teams in alphabetical order. It wasn’t rocket science, but it was a good little exercise in data manipulation.
Here’s a quick summary of the steps:
- Got the list of MLB teams from the web.
- Saved it to a text file.
- Read the file into a Python list.
- Removed newline characters.
- Sorted the list alphabetically.
- Printed the sorted list.
Pretty straightforward, right? Sometimes the simplest tasks are the most satisfying. Hope this helps you out with your own projects!
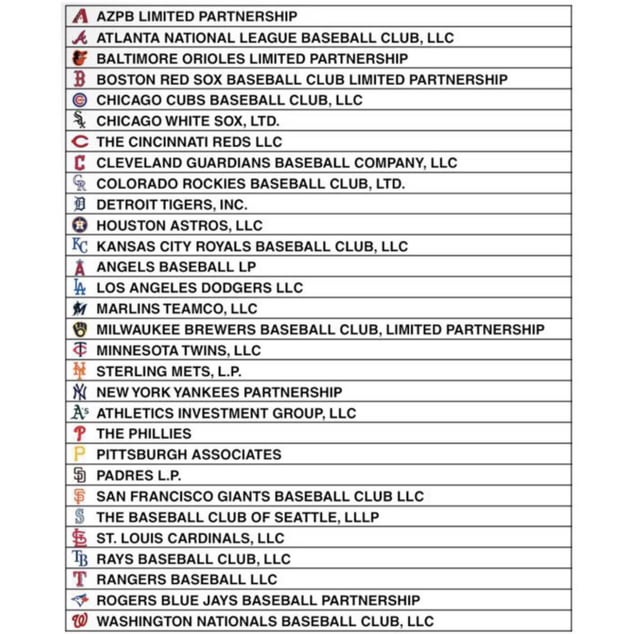
Pro-tip: You could easily adapt this to sort any list of strings, not just MLB teams. Just change the input data and you’re good to go!