Alright, so today I’m gonna walk you through my experience messing around with gronk
for pulling out specific bits of JSON related to height and weight. It was a bit of a journey, so buckle up.
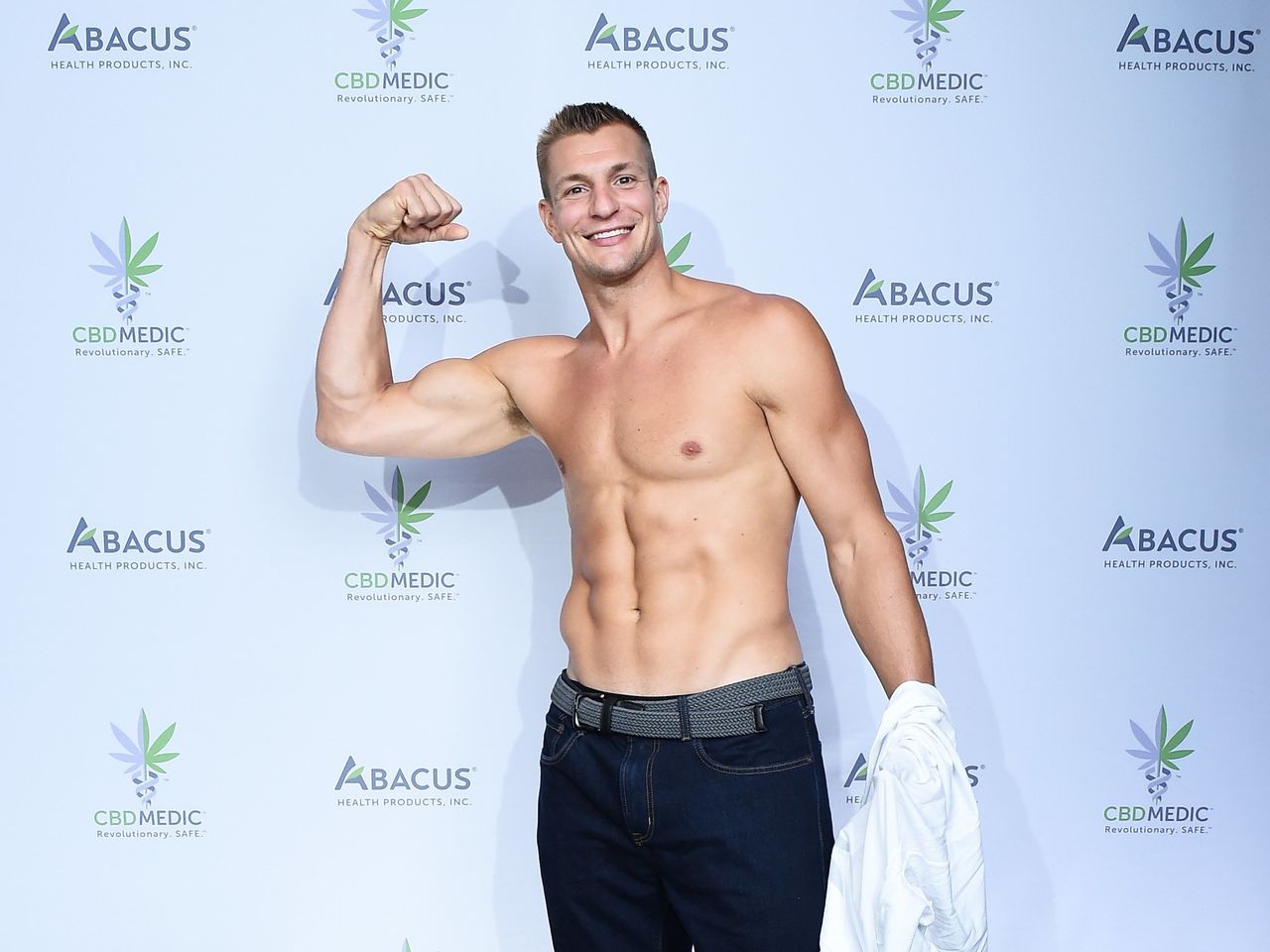
First off, I needed to grab some JSON data. I had this API endpoint that spat out a whole load of info, but all I cared about was the height and weight. So, I started with a simple curl
command to grab the JSON and pipe it into gronk
. Looked something like this:
curl "your_api_endpoint_here" gronk
This just throws the whole JSON structure at you, but in gronk
‘s indexed format. Easier to read, but still overwhelming. The key thing is that gronk
gives you these indexes you can then use.
Then came the fun part: figuring out the right gronk
incantation to get JUST the height. I knew the height was nested inside some objects in the JSON. After staring at the output for a while (and a LOT of trial and error), I found the path. Let’s say it was nested like this: . The command I ended up using was:
curl "your_api_endpoint_here" gronk grep "*"
This filters the output to only show lines containing that string. It’s not perfect, but it got me closer. I was seeing lines like *.height = "6ft 2in";
or *.height = 188;
I did the same thing to grab the weight. I searched for the string, for example:
curl "your_api_endpoint_here" gronk grep "*"
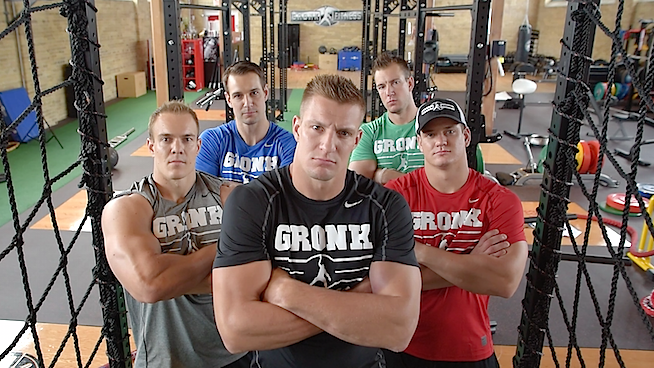
Okay, got both height and weight, but they were still mixed with the json.
prefix and the assignment. Time for some sed
magic. I wanted to clean up the output to ONLY show the actual value. So, I piped the output of the grep
command into sed
to strip away the unnecessary text.
For example, to clean the height:
curl "your_api_endpoint_here" gronk grep "*" sed 's/.= //g' sed 's/;//g'
The first sed
command s/.= //g
removes everything up to and including the equals sign. The second sed
command s/;//g
removes the semicolon at the end.
Then I did the same thing for weight to get the raw values.
At this point, I had the height and weight printed out, one after the other. But what if I wanted to store them into variables? Well, that’s where things got a little more shell-scripty.
I used command substitution to assign the output of each command to a variable. Like this:
height=$(curl "your_api_endpoint_here" gronk grep "*" sed 's/.= //g' sed 's/;//g')
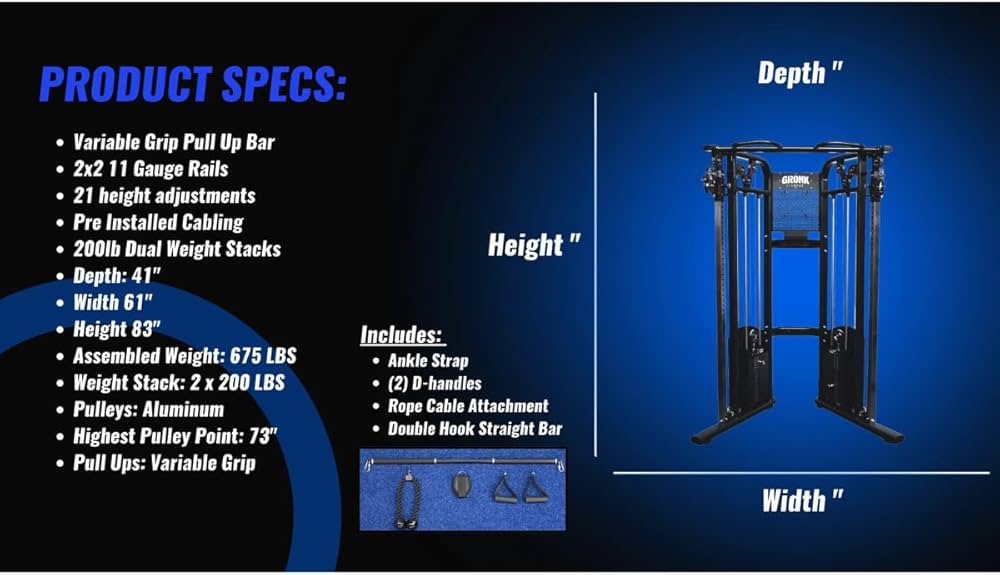
weight=$(curl "your_api_endpoint_here" gronk grep "*" sed 's/.= //g' sed 's/;//g')
Now, $height
and $weight
held the values I wanted! I could then use them in other parts of my script. For example, just to print them:
echo "Height: $height"
echo "Weight: $weight"
It wasn’t a walk in the park, there was a fair bit of tweaking and head-scratching, but it got the job done! Using gronk
with grep
and sed
is a powerful combo for pulling out specific pieces of JSON data when you don’t want to use a more heavy-duty tool like jq
.