Okay, so yesterday I was messing around trying to pull player stats from a Seattle Mariners vs. Houston Astros game. It sounded simple enough, right? Well, let me tell you, it was a bit of a journey.
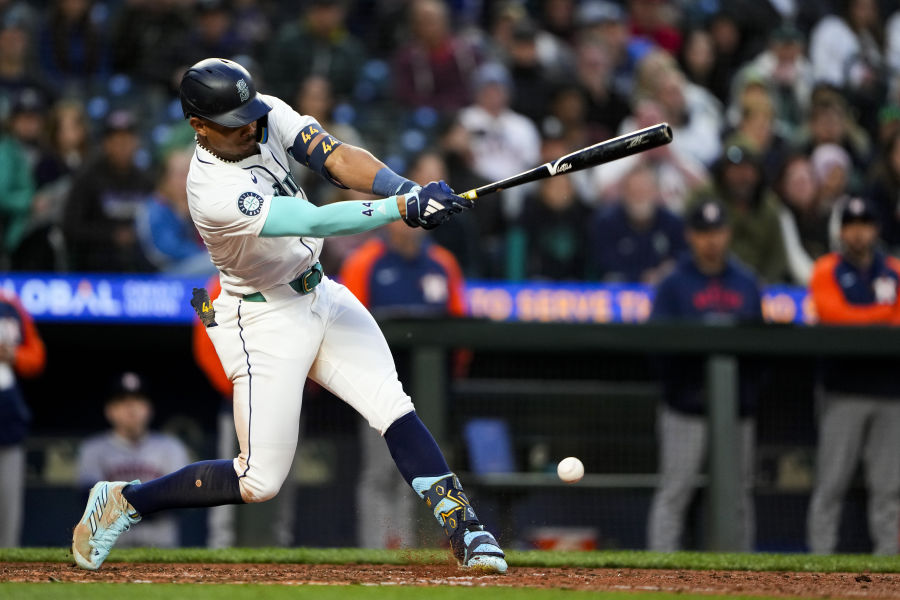
First things first: data source. I spent a good hour just googling around for a reliable source of baseball stats. There are a bunch of sites out there, but a lot of them are either paywalled or have really clunky APIs. Finally, I stumbled upon one that seemed promising, it was free to use and the data looked pretty clean.
Then came the coding part. I decided to use Python, ’cause that’s what I’m most comfortable with. I fired up my editor and started writing a script to fetch the data from the API. I used the ‘requests’ library to make the API calls.
It went something like this:
- Imported the ‘requests’ library.
- Crafted the API URL with the specific game ID for the Mariners vs. Astros match.
- Made a GET request to that URL.
- Checked if the request was successful (status code 200).
Sounds easy, right? But guess what? I kept getting errors! Turns out, I was missing an API key in my request header. After digging through the documentation, I finally figured out where to put it.
Next hurdle: parsing the JSON. The API returned a huge JSON blob with all sorts of info – game details, player stats, team stats, you name it. I needed to sift through all that and extract just the player stats for both teams.
I used the ‘json’ library to parse the JSON response into a Python dictionary. Then, I had to figure out the structure of the dictionary and navigate through nested lists and dictionaries to get to the player stats. It was like navigating a maze!
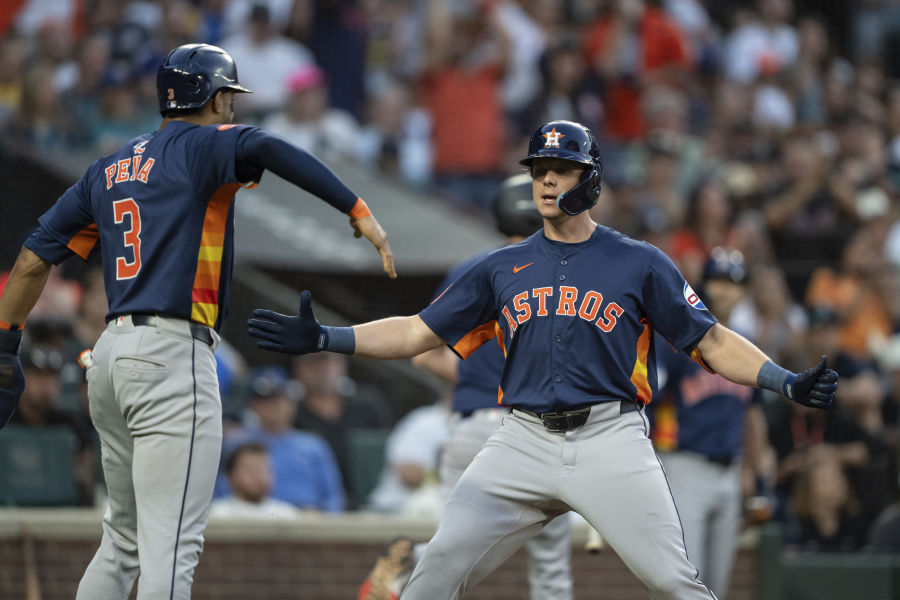
Data cleaning and formatting. Once I had the raw player stats, I needed to clean them up and format them nicely. There were missing values, weird abbreviations, and stats I didn’t even need.
I ended up doing a bunch of stuff like:
- Replacing missing values with zeros or ‘N/A’.
- Converting abbreviations to full names.
- Filtering out stats I didn’t care about (like fielding stats).
- Creating separate lists for Mariners and Astros players.
Finally, displaying the results. After all that, I wanted to display the player stats in a readable format. I decided to print them out in a simple table using the ‘tabulate’ library.
It was pretty straightforward:
- Created a table header with the column names (Player Name, At Bats, Runs, Hits, etc.).
- Iterated through the player lists and created rows for each player.
- Printed the table using ‘tabulate’.
And there you have it! After a few hours of coding, debugging, and data wrangling, I finally had a script that could pull and display player stats from a Mariners vs. Astros game. It wasn’t the most elegant solution, but it got the job done. Plus, I learned a ton about APIs, JSON parsing, and data cleaning along the way.
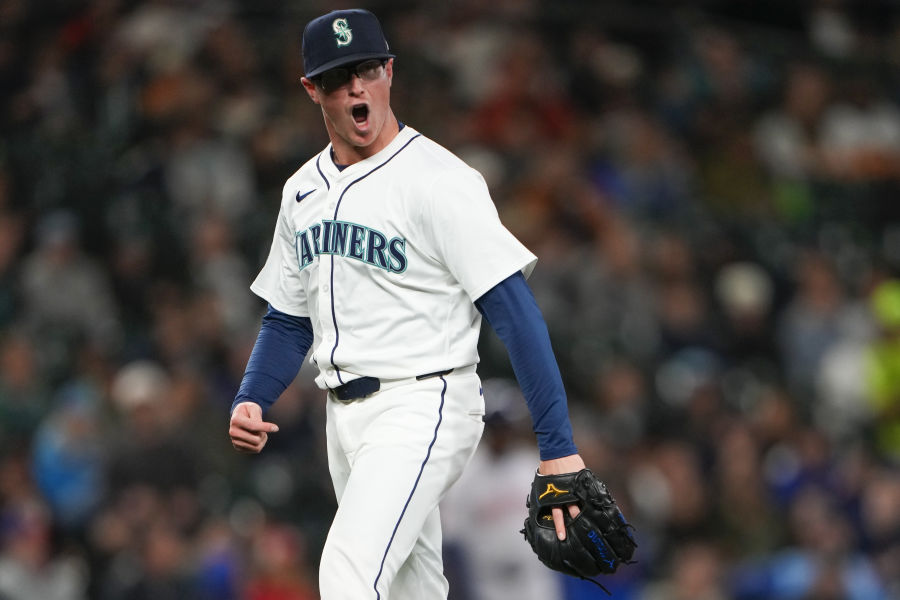
Lessons Learned:
- Always read the API documentation carefully.
- Be prepared to spend time cleaning and formatting data.
- Python is your friend.
Overall, it was a fun little project. I might try to automate it and build a little web app to display the stats in real-time. Who knows, maybe I’ll even become a baseball stats guru!
Future Improvements
I’m thinking of adding some features to this little project in the future. Maybe implement caching to avoid hitting the API too often, or even integrate some machine learning to predict player performance. The possibilities are endless!