Right, so I wanted to share a bit about this thing I wrestled with recently, involving jq and what we ended up calling the “gray” configs. It wasn’t anything fancy, just dealing with some messy setup files.
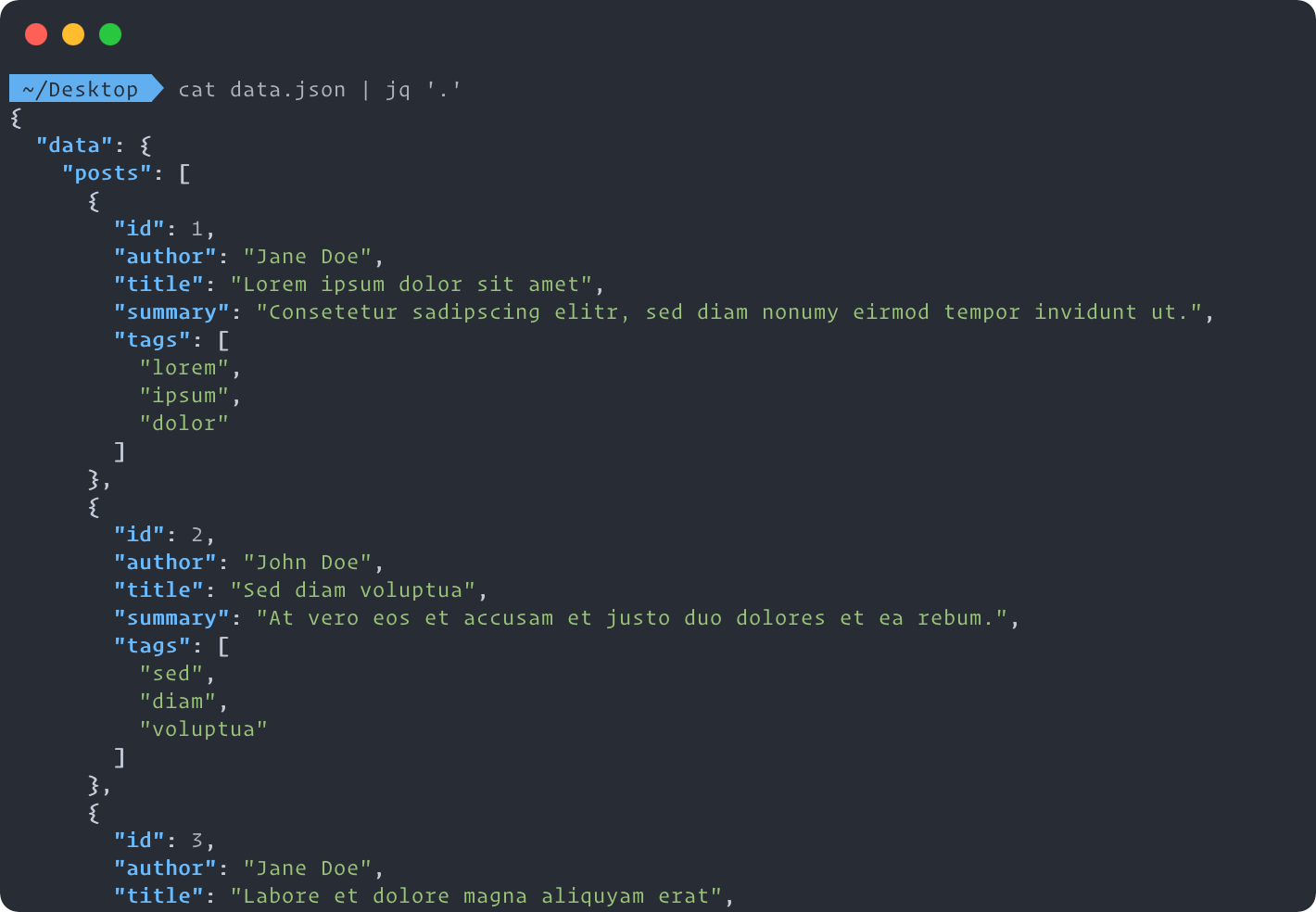
It started because we had these JSON files flying around that controlled who saw certain new features on a project. Like, not everyone, just a handful of test users or maybe a specific region. A sort of basic, manual “gray” deployment, you know? The problem was, these JSONs were becoming a real pain to manage.
The Initial Mess
At first, I was just opening them in a text editor. Find the user ID, change a `false` to a `true`, save, done. Simple. But then things got complicated.
- The files got bigger. Nested structures appeared out of nowhere.
- We needed to enable features not just by user ID, but sometimes by email domain, or based on some other random flag someone added.
- Manually checking who was in the “gray” group became a nightmare. You’d scroll and scroll, easily miss things.
I tried using simple command-line tools, like `grep`. You know, searching for `”userId”: “123”` or whatever. But `grep` doesn’t understand the structure. It would match stuff inside comments, or parts of other values. It was messy and unreliable.
Finding jq
I remembered someone mentioning jq a while back for command-line JSON stuff. Honestly, I’d avoided it because it looked complicated at first glance. But the manual editing was getting ridiculous, so I decided to give it a proper try.
First steps were easy enough. Just grabbing simple values. If I had a file like `*`, I could do something like `jq ‘.version’` to see the version. Okay, that works.
Then I figured out how to dig into arrays or nested objects. Like `jq ‘.users[0].name’` to get the first user’s name. Still pretty basic.
Tackling the “Gray” Part
The real challenge was filtering based on those conditions for our “gray” rollout. I needed to find all users who had `featureXEnabled: true` or belonged to a certain group.
This is where jq started to shine, after some head-scratching. The `select()` function was the key. I spent a good chunk of time just experimenting on the command line.
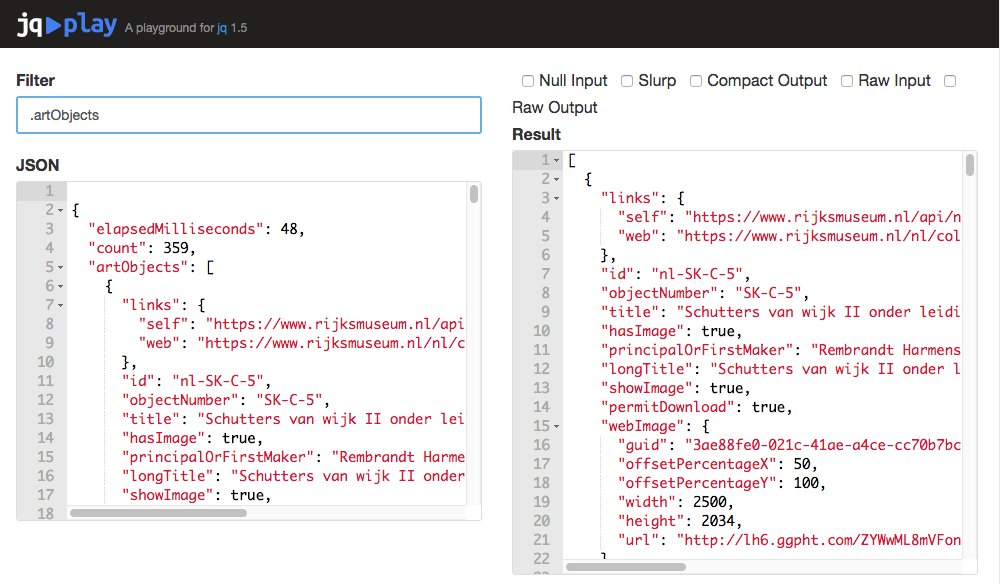
I started doing things like this:
jq '.users[] select(.featureXEnabled == true)' *
That command would spit out only the user objects where `featureXEnabled` was true. Suddenly, I could easily see who was supposed to be in the test group!
It got more complex when I needed multiple conditions. Combining `select()` calls, using `and`/`or`. The syntax took a bit to get used to, felt weird at first. Lots of trial and error, checking the `jq` manual pages online constantly.
I ended up writing small shell scripts that used `jq` to:
- List all users currently in the “gray” group for a specific feature.
- Check if a particular user ID was enabled.
- Even generate smaller JSON files containing only the enabled users, which was sometimes useful for other tools.
Looking Back
So yeah, that’s how I started using jq for managing those “gray” configurations. It wasn’t some super advanced technique, just applying a tool to solve a practical, annoying problem.
It definitely beat manual editing or unreliable `grep` commands. Made things much faster and safer. Still think the original JSON structure was way too complicated, but hey, `jq` helped me tame it, mostly. It’s become a standard part of my toolkit now whenever I have to wrangle JSON on the command line.