Alright, so I’m gonna walk you through how I tackled building a classic leaderboard in Creator. It was a bit of a journey, but hey, that’s what makes it fun, right?
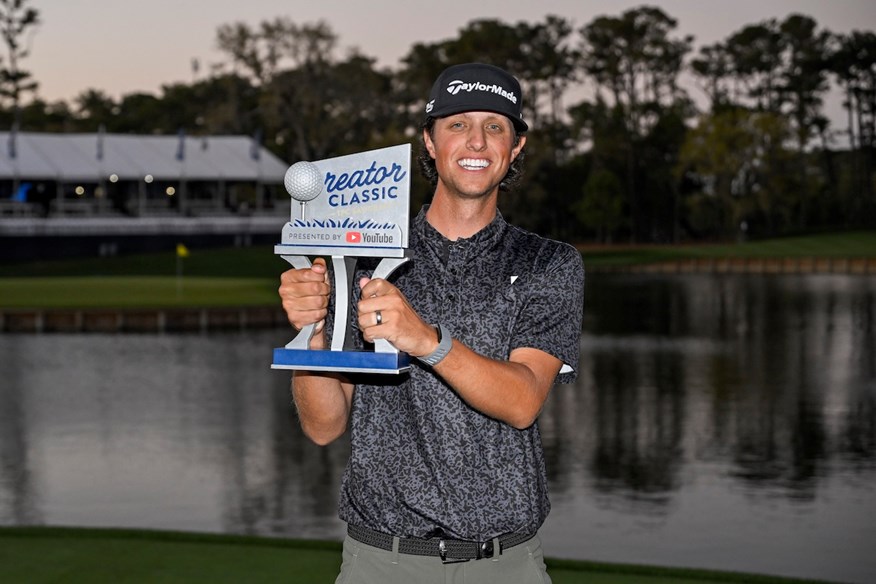
First off, I fired up Creator and created a new project. Kept it simple, you know? Just the basics to get started. I decided on the visual style – went for something clean and straightforward, easy to read at a glance.
Next, the data! This is where things got a little more interesting. I needed a way to store player scores. I ended up using a simple JSON file for persistence, nothing fancy. I wrote some functions to read and write to this file. Think of it like this:
- LoadData(): Reads the JSON file and loads the score data into a list of player objects.
- SaveData(): Takes the player data list and writes it back to the JSON file.
- AddScore(playerName, score): Adds a new score for a player or updates the existing score if the player is already on the leaderboard.
Now, the UI. I dragged in a Canvas, added a ScrollView for the leaderboard entries, and created a prefab for each entry. The prefab had a Text component for the player’s name, another for their score, and maybe a rank number. I used a simple List to hold instances of the entries.
Here’s the tricky part: dynamically populating the leaderboard. I wrote a function called `UpdateLeaderboard()` that did the following:
- Cleared the existing leaderboard entries.
- Loaded the score data using `LoadData()`.
- Sorted the data based on the score in descending order (highest score first).
- Iterated through the sorted data and instantiated a new leaderboard entry prefab for each player. I set the text of each entry to display the player’s name, score, and rank.
- Added the new entry to the ScrollView’s content.
To make it interactive, I added a simple input field and a button. Players could enter their name, submit their score, and then the leaderboard would update. The button’s `onClick` event was connected to a function called `SubmitScore()`. This function grabbed the player’s name and score from the input fields, called `AddScore()` to update the data, and then called `UpdateLeaderboard()` to refresh the display.
Of course, there were a few bugs along the way. I spent some time debugging why the leaderboard wasn’t updating correctly. Turns out, I forgot to sort the data before updating the UI – rookie mistake! Once I fixed that, everything started working smoothly.
Finally, I added some polish. A bit of styling, maybe some animations, just to make it look a little nicer. And that was it! A simple, classic leaderboard in Creator. Nothing too fancy, but it gets the job done.
Learned a lot, had some fun, and now I’ve got a reusable leaderboard component for future projects. Not bad, eh?
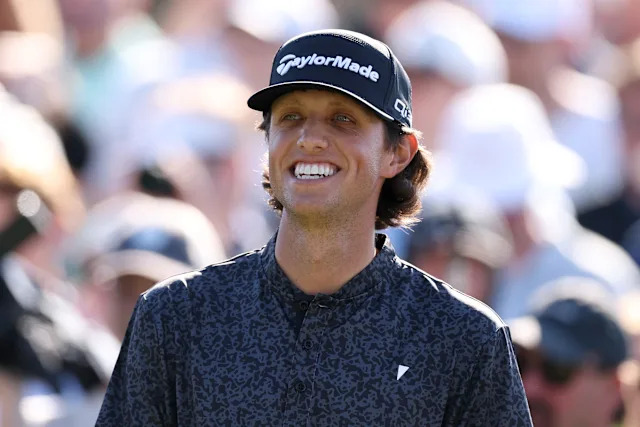